9 Must-know Patterns for Writing Clean Code With Python
Python developers still need to learn python best practices and design patterns in order to write clean code. Here are the 9 Must-know Patterns for Writing Clean Code with Python.
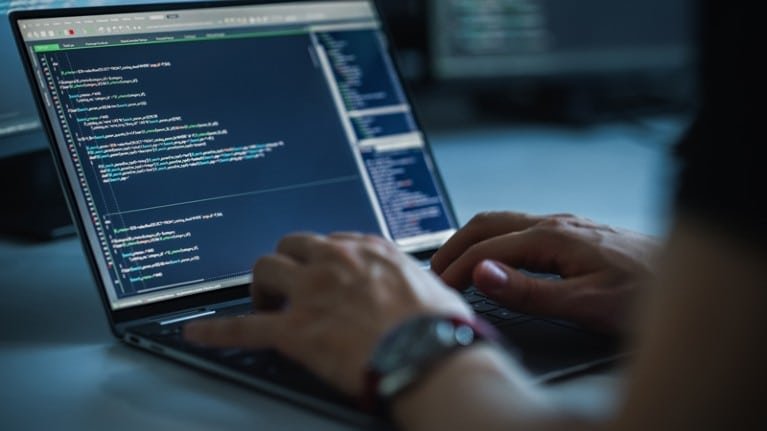
Python is one of the most elegant and clean programming languages available, yet having a beautiful and clean syntax does not imply creating clean code. Surprisingly, Python has surpassed Java in the list of top programming languages and is now the most learned! In this blog, we’ve compiled a list of must-know patterns for Writing Clean Code with Python.
It is the second most used language after JavaScript and is gradually edging out of the competition to take the top spot.
It is widely used in a variety of disciplines, including web development using popular frameworks such as Django and Flask, web scraping, automation, system administration, DevOps, testing, network programming, data analysis, data science, machine learning, and artificial intelligence.
Table of Contents
What is a Clean Code?
This quote from Bjarne Stroustrup, the creator of the C++ programming language, elucidates what clean code entails:
“I like my code to be elegant and efficient. The logic should be straightforward to make it hard for bugs to hide, the dependencies minimal to ease maintenance, error handling complete according to an articulated strategy, and performance close to optimal so as not to tempt people to make the code messy with unprincipled optimizations. Clean code does one thing well.”
We may derive some of the characteristics of clean code from the quote:
- Clean code is focused. Each function, class, or module should do one thing and do it well.
- Clean code is easy to read and reason about. According to Grady Booch, author of Object-Oriented Analysis and Design with Applications: clean code reads like well-written prose.
- Clean code is easy to debug.
- Clean code is easy to maintain. That is it can easily be read and enhanced by other developers.
- Clean code is highly performant.
9 Must-Know Patterns for Writing Clean Code with Python:
1. Use descriptive names that are lengthy and simple to read.

As a result, there will be no need to write needless remarks, as seen below:
# Not recommended
# The au variable is the number of active users
au = 105
# Recommended
total_active_users = 105
2. Use descriptive intention to reveal names.
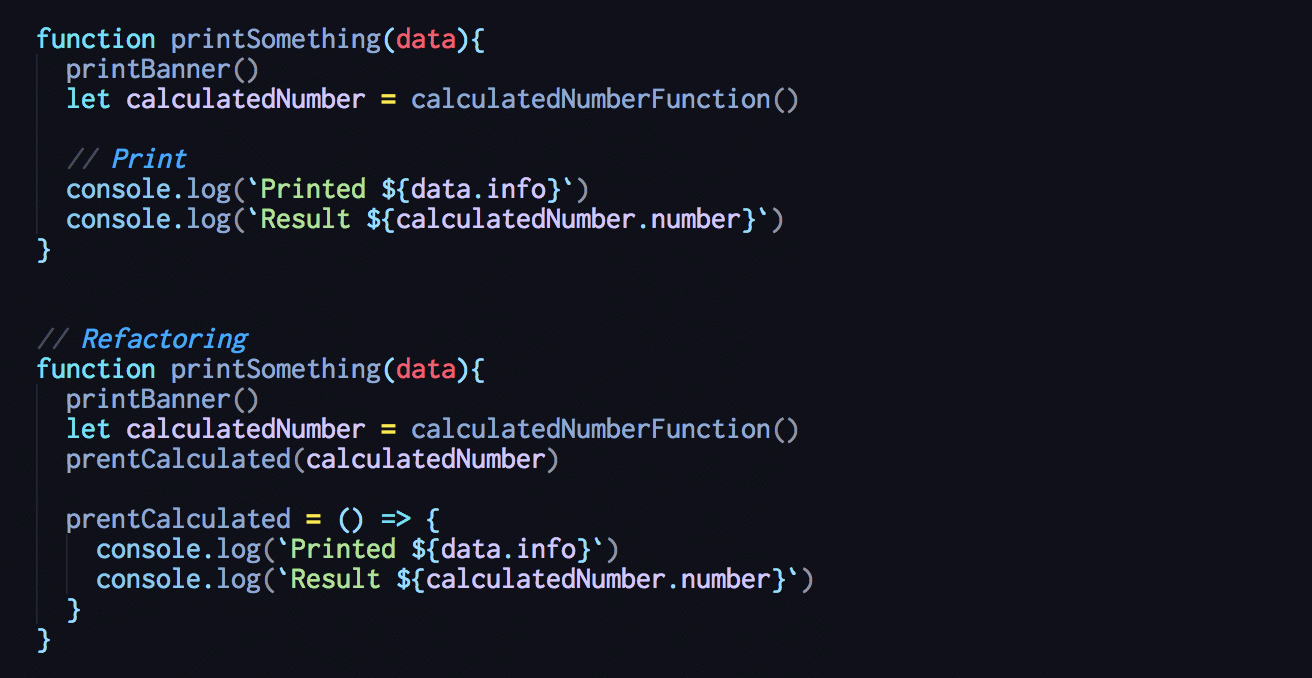
From the name, other developers should be able to deduce what your variable holds. In a nutshell, your code should be simple to read and understand.
# Not recommended
c = [“UK”, “USA”, “UAE”]
for x in c:
print(x)
# Recommended
cities = [“UK”, “USA”, “UAE”]
for city in cities:
print(city)
3. Avoid using ambiguous shorthand.

A variable’s name should be lengthy and descriptive rather than short and confusing.
# Not recommended
fn = ‘John’
Ln = ‘Doe’
cre_tmstp = 1621535852
# Recommended
first_name = ‘JOhn’
Las_name = ‘Doe’
creation_timestamp = 1621535852
4. Always use the same vocabulary.
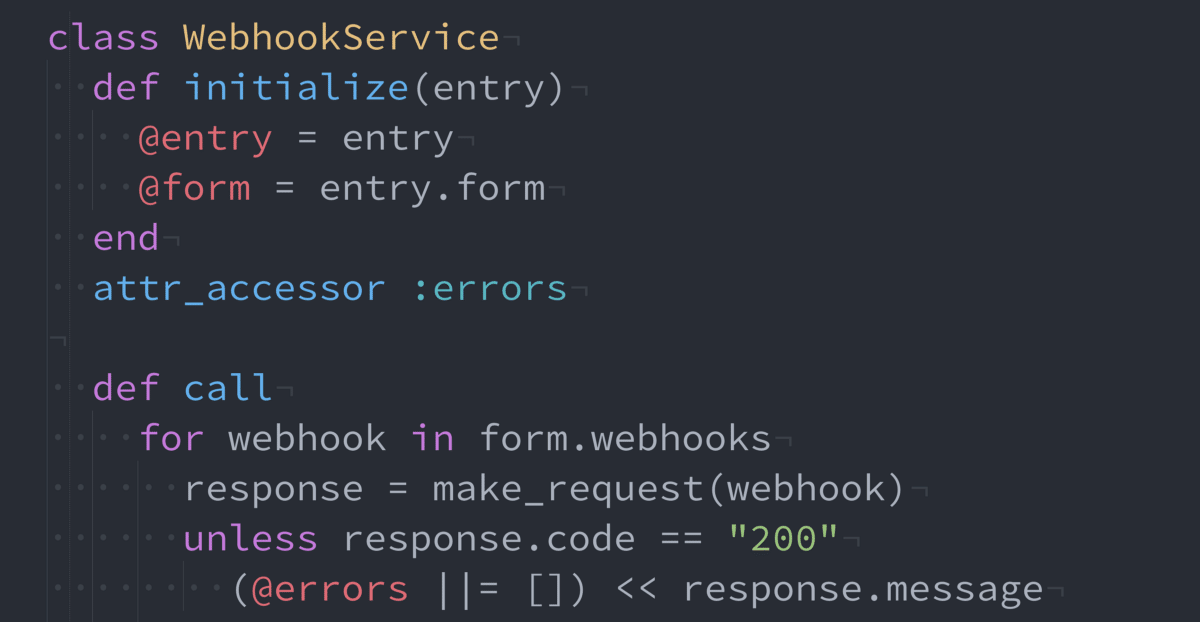
Maintain consistency in your naming convention. Maintaining a consistent naming convention is critical to avoiding misunderstanding when working with other developers on your code. This is true when it comes to naming variables, files, functions, and even directory structures.
# Not recommended
client_first_name = ‘John’
customer_last_name = ‘Doe;
# Recommended
client_first_name = ‘John’
client_last_name = ‘Doe’
Also, consider this example:
#bad code
def fetch_clients(response, variable):
# do something
pass
def fetch_posts(res, var):
# do something
pass
# Recommended
def fetch_clients(response, variable):
# do something
pass
def fetch_posts(response, variable):
# do something
pass
5. Don’t use magic numbers.

Magic numbers are numbers that exist in code but have no meaning or explanation because they have specific, hard-coded meanings. Typically, these numbers exist as literals in several places in our code.
import random
# Not recommended
def roll_dice():
return random.randint(0, 4) # What is 4 supposed to represent?
# Recommended
DICE_SIDES = 4
def roll_dice():
return random.randint(0, DICE_SIDES)
6. Be consistent with your function naming convention.
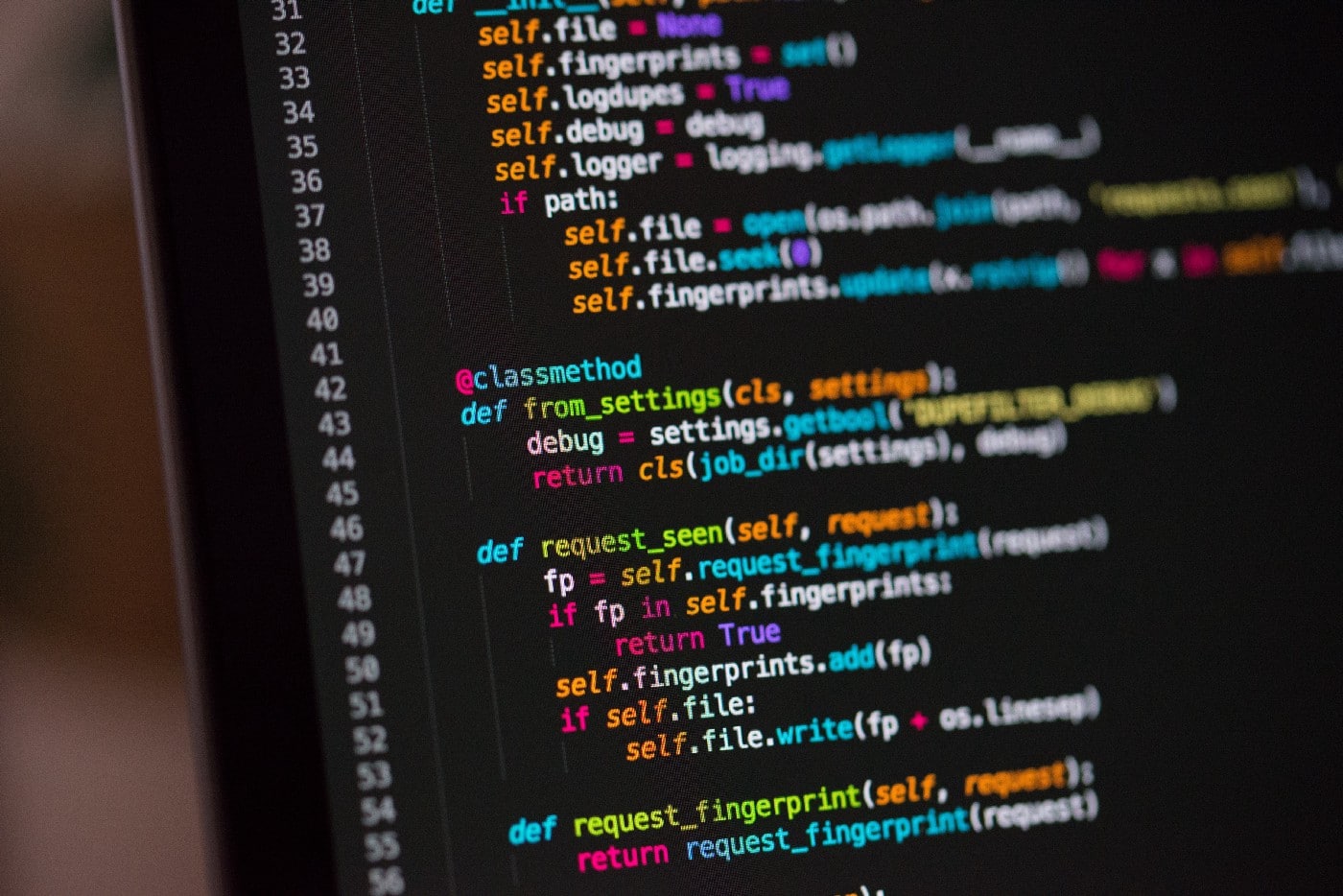
When naming functions, as with variables, follow a naming standard. Other developers might be confused if you used various naming standards.
# Not recommended
def get_users():
# do something
Pass
def fetch_user(id):
# do something
Pass
def get_posts():
# do something
Pass
def fetch_post(id):
# do something
pass
# Recommended
def fetch_users():
# do something
Pass
def fetch_user(id):
# do something
Pass
def fetch_posts():
# do something
Pass
def fetch_post(id):
# do something
pass
7. Functions should do one thing and do it well.

Write brief, straightforward functions that do a single thing. A good rule of thumb to remember is that if your function name contains the word “and,” you should consider splitting it into two functions.
# Not recommended
def fetch_and_display_users():
users = [] # result from some api call
for user in users:
print(user)
# Recommended
def fetch_usersl():
users = [] # result from some api call
return users
def display_users(users):
for user in users:
print(user)
8. Do not use flags or Boolean flags.
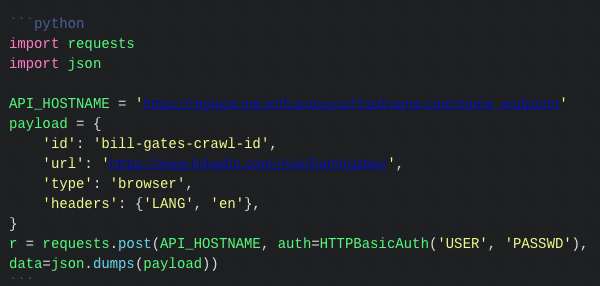
Variables that carry a boolean value — true or false — are known as boolean flags. These flags are supplied to a function, which uses them to define its behavior.
text = “Python is a simple and elegant programming language.”
# Not recommended
def transform_text(text, uppercase):
if uppercase:
return text.upper()
else:
return text.lower()
uppercase_text = transform_text(text, True)
lowercase_text = transform_text(text, False)
# Recommended
def transform_to_uppercase(text):
return text.upper()
def transform_to_lowercase(text):
return text.lower()
uppercase_text = transform_to_uppercase(text)
lowercase_text = transform_to_lowercase(text)
9. Do not add redundant context.
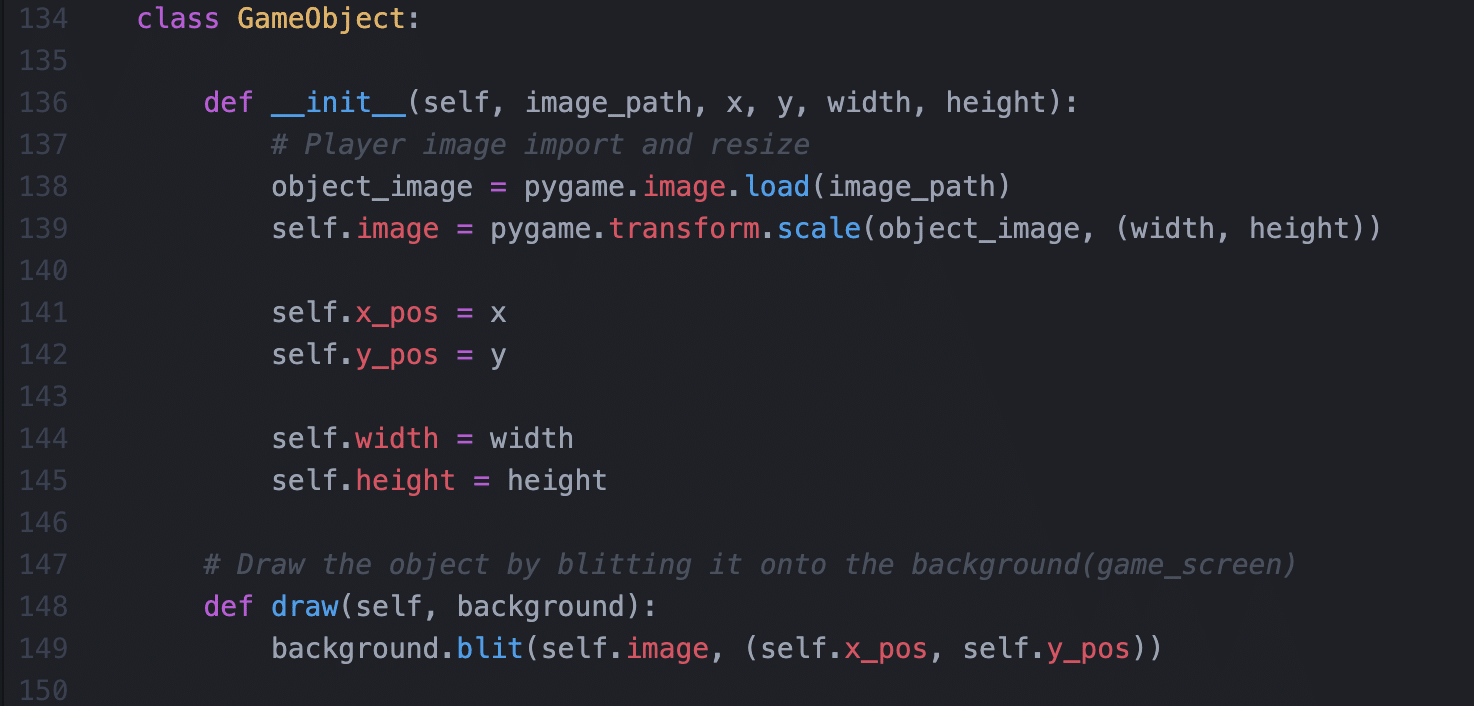
This can happen while dealing with classes and adding extra variables to variable names.
# Not recommended
class Person:
def __init__(self, person_username, person_email, person_phone, person_address):
self.person_username = person_username
self.person_email = person_email
self.person_phone = person_phone
self.person_address = person_address
# Recommended
class Person:
def __init__(self, username, email, phone, address):
self.username = username
self.email = email
self.phone = phone
self.address = address
Split your logic into multiple files or classes called modules to keep your code tidy and manageable. In Python, a module is just a file with the.py suffix. And each module should be laser-focused on performing one thing well. You can adhere to basic object-oriented — OOP concepts such as encapsulation, abstraction, inheritance, and polymorphism.
Writing clean code has several benefits, including increased software quality, code maintainability, and the elimination of technical debt. Hope this article on must-know patterns for writing clean code with Python was helpful to you.
Also Checkout: Top 8 Airtable Alternatives For You To Try